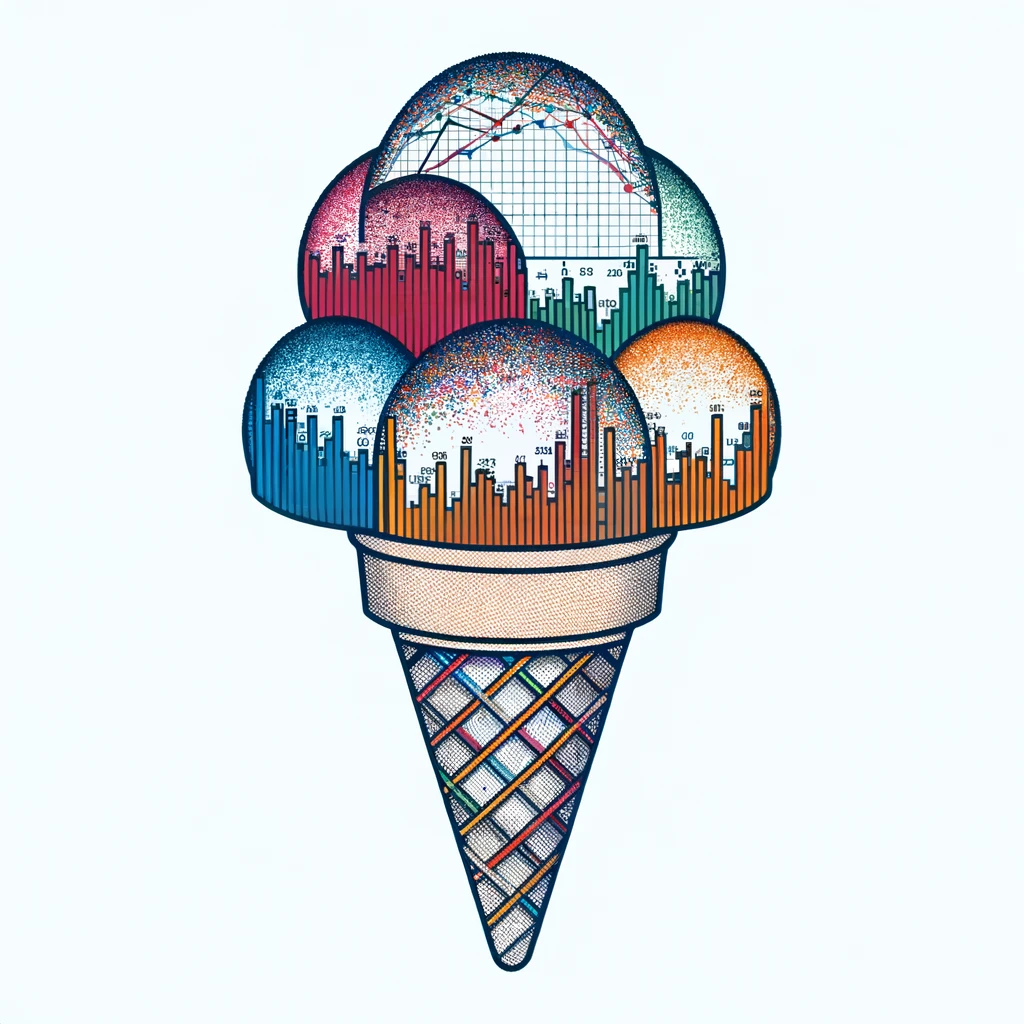
icecream debugger
Intro
There’s a wide gap between print()
debugging and setting up good logging and observability.
icecream is a very easy debugging tool that’s easy to use. I’ll show an example of how to use it to add some debugging information without much effort.
misc_devtools_icecream.ipynb
Install icecream first¶
Uncomment the following line
In [1]:
# %pip install icecream
Load some data¶
In [2]:
import bql
import xgboost as xgb
import plotly.express as px
In [3]:
daterange = 29 # days
security = "IBM US Equity"
basics_query = f"""get(
px_last
) for(
['{security}']
) with(
dates=range(-{daterange}d, 0d),
fill=prev,
currency=USD
)"""
bql_svc = bql.Service()
response = bql_svc.execute(basics_query)
base_df = bql.combined_df(response)
# Reset the index: bql's combined_df returns ID as a sole index.
base_df = base_df.reset_index()
base_df["date_ordinal"] = base_df["DATE"].apply(lambda x: x.toordinal())
Load icecream¶
Icecream is a better print debugger. In practice, I like to use loggers, but this is fine.
In [4]:
from icecream import ic
ic.configureOutput(includeContext=True, contextAbsPath=False)
def warn(s):
print(s)
ic.configureOutput(outputFunction=warn)
Using the XGBoost example¶
Modify this to add some variables
In [5]:
def my_entrypoint():
xg_df = base_df.copy()
# IC: Print the last row
ic(len(xg_df))
xg_df["month"] = xg_df["DATE"].dt.month
xg_df["day_of_week"] = xg_df["DATE"].dt.dayofweek
train_df = xg_df.iloc[:-21]
test_df = xg_df.iloc[-21:]
X_train = train_df[["month", "day_of_week"]]
y_train = train_df["px_last"]
xmodel = xgb.XGBRegressor(
n_estimators=100, learning_rate=0.1, objective="reg:squarederror"
)
xmodel.fit(X_train, y_train)
xg_df.loc[test_df.index, "xgpredicted_px_last"] = xmodel.predict(
test_df[["month", "day_of_week"]]
)
# IC: Print the last row
ic(xg_df.iloc[-1])
px.line(xg_df, x="DATE", y=["px_last", "xgpredicted_px_last"])
In [6]:
my_entrypoint()
ic| 752635400.py:5 in my_entrypoint()- len(xg_df): 30
ic| 752635400.py:26 in my_entrypoint() xg_df.iloc[-1]: index 29 ID IBM US Equity DATE 2023-06-27 00:00:00 px_last 0.027987 date_ordinal 738698 month 6 day_of_week 1 xgpredicted_px_last 0.28129 Name: 29, dtype: object